I cannot live without these VueUse utils
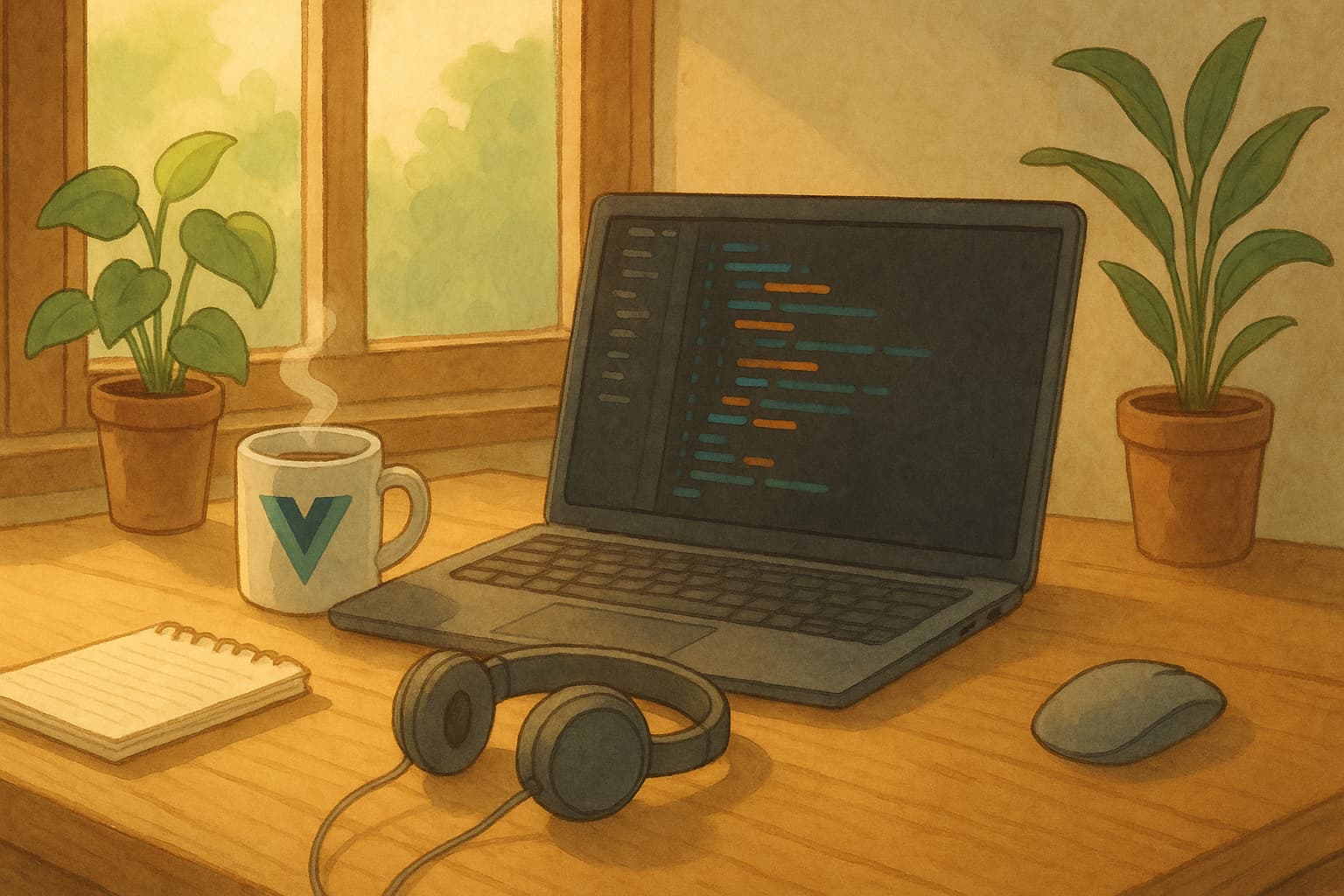
I've been using VueUse for ages in my projects, if you are writing a reusable util, chances are you can find it in VueUse.
If you're a vue dev for some time, you probably know what I'm talking about. Some of these tools are so powerful together, you'll wonder how you ever lived without them.
Some might argue these are just simple abstractions, but thats the whole point of vueuse
useDateFormat and useTimeAgo
The useDateFormat composable has basically solved all of my date formatting needs. I cannot stress enough how useful this is, it accepts a simple format you are expecting the date to be in and it will return the date in the correct format.
useTimeAgo is just as useful, returns you those 2 days ago
strings.
refDebounced
Want to add a delay to your search so it starts after the user stops typing? Use refDebounced. The example might be a bit confusing if you're new to it
Here's a simple example:
<script setup lang="ts">
import { refDebounced } from '@vueuse/core'
const input = ref('')
const searchDebounced = refDebounced(input, 300) // 300ms delay
watch(searchDebounced, async (value) => {
const res = await fetch(`/api/search?q=${value}`)
})
</script>
Link refDebounced
useDropZone, useFileDialog and useObjectUrl
These three together are great when you're building a drag-and-drop file uploader.
I usually use useDropZone for a drop area and add a button in the middle to open the file dialog with useFileDialog. Then, I use useObjectUrl to show a preview of the file before it's uploaded.
Here's how I use it in most of my projects.
<template>
<div class="flex items-center gap-2">
<UAvatar
:src="model || undefined"
icon="i-lucide-upload"
/>
<UButton
size="xs"
type="button"
:label="model ? 'Change' : 'Upload'"
@click="() => open()"
/>
<UButton
v-if="model"
label="Remove"
@click="removeImage"
/>
</div>
</template>
<script setup lang="ts">
import { useFileDialog, useObjectUrl } from '@vueuse/core'
const model = defineModel<string | undefined>()
const { files, open, onChange } = useFileDialog({
accept: 'image/*',
multiple: false,
})
const emit = defineEmits<{
'file-selected': [file: File | null]
}>()
onChange(() => {
const file = files.value?.[0]
if (file) {
const objectUrl = useObjectUrl(file)
model.value = objectUrl.value
emit('file-selected', file)
}
})
const removeImage = () => {
model.value = ''
emit('file-selected', null)
}
</script>
createSharedComposable
I found out about this when I was looking through Nuxt UI's code, and it blew my mind. I didn't know you could do this, and it kind of changed how I write Vue code. It shares the data, variables, and state when you use it in different components.
Link: createSharedComposable
useElementVisibility
It shows you if the element is visible on the screen or not. I mostly use this when I need animations that start when you scroll to them on website landing pages.
Link: useElementVisibility
useBreakpoints
When CSS isn't enough, use useBreakpoints to get the user's current breakpoint. It comes with a bunch of presets, and I mostly use the breakpointsTailwind
ones.
I use this when I need to add dynamic props to components based on the screen sizes.
useWebWorker and useWebWorkerFn
If you've ever worked with web workers, you know how annoying it is to get them working with different tooling and frameworks. I found both of these composables by accident, and they've become my go-to when using web workers. Most of the web worker code in https://minipic.app uses these tools.
Link: useWebWorker
Link: useWebWorkerFn
useClipboard
The main reason I use this is that it gives you a "copied" state, and the "copy" function is async. It also has a fallbacl for older browsers that don't support the latest features.
Link: useClipboard
useScriptTag
This makes working with external scripts super simple. It has events for "load" and even has a manual mode to control the script tag to load them when you want.
Link: useScriptTag
useInfiniteScroll
If you want to add infinite scroll, this tool is pretty useful. It automatically detects when the user has reached the bottom of the container and loads more data.
Works really well with useVirtualList.
Link: useInfiniteScroll
useEventSource
I was learning about SSE, and it was really confusing. Since not many people use it, it was hard to find info. This tool makes it super easy to listen to events from the server in real-time.
I've only used this in one project, but it made working with SSE easy for me.
useWebSocket
If you've ever worked with websockets, you have to handle a lot of cases. This util handles all of them.
Link: useWebSocket